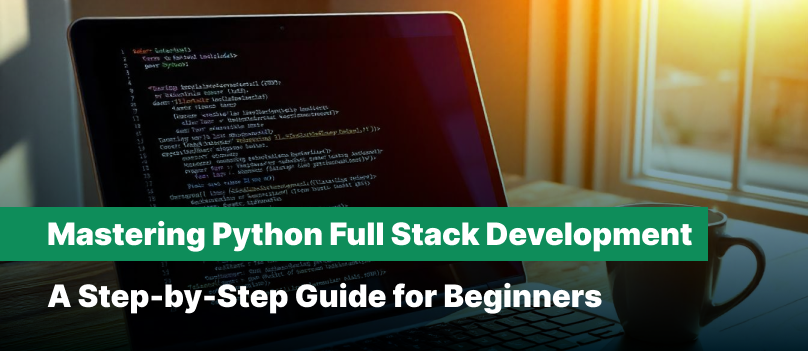
“Mastering Python Full Stack Development: A Step-by-Step Guide for Beginners”
- Date January 13, 2025
Introduction
Today the world and technology are very entwined to a point that one cannot contemplate on living life without this major aspect. Between the apps we deploy daily and the websites we navigate instinctively lies an invisible layer of genius—the handiwork of Full Stack Developers. These modern technologists are akin to a Swiss Army knife in the programming universe, equally at ease crafting visually appealing front-end interfaces as they are engineering robust, back-end infrastructures. They are the architects of today’s digital experiences.
Imagine building a sleek, modern website: the fluid animations, intuitive navigation, and eye-catching layouts draw users in. Now, pair that with a seamless backend—the invisible force handling data, transactions, and user authentication with the precision of a Swiss watch. Together, these aspects merge into a singular, elegant product. TIt means that The physical and the metaphysical: this is where Full Stack Development really comes alive.
And the essence of this craft is Python, an actually well-known programming language due to the simplicities of its uses and active across almost all industry types. Python means—an enabler that enables the Programming novices to shape part of the Full-Fledged Application Development machinery. In this guide, you’ll embark on a journey through the layered world of Python Full Stack Development, gaining insight into the foundational building blocks and practical know-how required to create real-world applications.
Demystifying Full Stack Development: The Big Picture
Before diving into the mechanics, let’s decode what “Full Stack Development” truly entails. Is it merely a fusion of front-end and back-end development? Or does its essence run deeper?
A Full Stack Developer does it all—they design the user-facing visual experience (front-end) and engineer the functionality that drives it behind the scenes (back-end). In technical terms:
–
The front-end is what users see and interact with: of rooms, tones, buttons, forms and signs. It is run by HTML, CSS as well as JS. The back-end performs its processes quietly in the background and takes care of encoding databases, Application Programming Interfaces (APIs), business logics and server-side procedures. I
Python recognizes a variety of data structures including dictionaries, lists and sets and therefore one can model a real word theme and concept well. It ensures flawless communication between the user and the underlying system.
To simplify, think of a luxury car: the stylish, ergonomic cabin represents the front-end, while the roaring engine and intricate transmission represent the back-end. The driver feels the impact of both. In this analogy, a Full Stack Developer is the automotive engineer who designs the cabin and tunes the engine to create a harmonious experience.
Python plays an instrumental role in providing developers with the tools to build every layer of this stack. But why is Python the preferred language? Let’s explore.
Why Does Python Reign Supreme in Full Stack World?
Covering the whole spectrum of application, programming languages are as numerous as they are diverse. So, what sets Mr. Python out from the rest in the highly saturated Full Stack Development marketplace? The answer is that the language is both simple and mighty and it also exists in a rich ecosystem .
1. Easy to Read and Basic Word Order
Python even has the natural English to script like appearance which offers it to be the best language that is both easy to learn, and highly functional. To the beginner getting into the development level, Python makes it easier to begin while at the same time providing the depth required in the development process.
Ex: # Python syntax is straightforward, almost readable as English:
user = “Alex”
print(f”Hello, {user}!”)
2. Frameworks That Cover Every Need
Python’s appeal skyrockets with its rich ecosystem of frameworks. On the back-end, frameworks like Django and Flask serve as invaluable tools:
Django: A robust, all-inclusive framework that handles everything from authentication to database queries.
Flask: A minimalist framework that allows developers to build highly customized applications.
On the front-end? Python isn’t the star player there (that’s the realm of HTML, CSS, and JavaScript), but it integrates brilliantly with front-end frameworks, creating a unified full stack workflow.
3. Perfect for Rapid Prototyping
So you want to grab a quick prototype or build a basic functional product in hours? Python lets you do so easily—With the help of libraries, what you may take days in doing, similar task can be done in hours in Python.
4. Realms of Employment and Employment Duration
The demand for people who know Python programming language is enormous. The projection for 2025 shows that there is continued dominance of Python in all aspects, right from web to Machine learning. Employers seek individuals who have mastery of Python in full stack positions where skills and artistry intersect. Python is not just learning sequence of commands, you are opening a very large area of doors for yourself.
Building Blocks of Python Full Stack Development: Step-by-Step
To master Python Full Stack Development, you need to tackle it layer by layer, just as you would assemble a skyscraper from a solid foundation upward.
Layer 1: Python Fundamentals—The Developer’s Ground Zero
Every great application begins with a single line of code, and for Python, that first line is often a revelation. Before venturing into frameworks and databases, you’ll need strong fundamentals.
Variable and Data Mastery
Variables are the containers that house your data. Python’s simplicity lets you focus on handling these blocks creatively.
# Variables representing user data
username = “CodeMaster”
age = 25
is_logged_in = True
Python supports a medley of data structures like dictionaries, lists, and sets, allowing you to model complex real-world concepts easily.
shopping_cart = {
“id”: 101,
“items”: [“Laptop”, “Headphones”, “Charger”],
“total”: 599.99,
“discounted”: False
}
Control Flow: Making Decisions Programmatically
By introducing efficient control mechanisms like if-else and loops, Python enables developers to create logic that adapts dynamically to different inputs.
def age_category(age):
if age < 18:
return “Minor”
elif age <= 65:
return “Adult”
else:
return “Senior Citizen”
This isn’t just syntax. Control-flow mastery lays the groundwork for coding dynamic behavior.
Object-Oriented Programming (OOP) Paradigm
OOP lets you encapsulate behavior and reuse code effectively. This is pivotal as applications grow larger.
class User:
def __init__(self, name, email):
self.name = name
self.email = email
self.is_active = False
def activate_account(self):
self.is_active = True
return f”{self.name}’s account is now active.”
Layer 2: Front-End Wizardry
Full Stack Developers aren’t isolated to Python alone. While Python powers the backend, the real magic of user interaction resides in front-end technologies.
HTML and CSS Are Non-Negotiable
Your first task is understanding HTML’s structure and CSS’s styling power. Working together, they create the visual blueprint of your application.
<!– HTML Example –>
<html>
<head><title>Welcome Page</title></head>
<body>
<h1>Welcome to the Portal</h1>
<p>Your digital solutions start here!</p>
</body>
</html>
/* CSS Styling */
h1 {
color: #007BFF;
text-align: center;
}
Add Dynamism with JavaScript
While Python rules server-side logic, on the client-side, JavaScript is king. Imagine adding interactive features to your web page—modals, animations, or toggles.
function showAlert() {
alert(“Welcome to Full Stack Development!”);
}
Layer 3: Back-End Brilliance
Now comes Python’s domain—the “engine room” of your application.
Why Django Reigns Supreme
Django simplifies backend development by abstracting complex, repetitive tasks. With its ORM (Object-Relational Mapping), developers avoid writing raw SQL.
# Adding a database model in Django
from django.db import models
class Product(models.Model):
name = models.CharField(max_length=100)
price = models.DecimalField(max_digits=10, decimal_places=2)
inventory = models.IntegerField()
Django automatically turns this model into a relational database table!
Flask for Lightweight Applications
Flask provides unparalleled flexibility for low-complexity or niche projects.
from flask import Flask
app = Flask(__name__)
@app.route(‘/’)
def homepage():
return “Welcome to Flask!”
Layer 4: Database Integration
A database breathes life into your applications. Python frameworks like SQLAlchemy or Django ORM streamline database management.
SQLAlchemy Example
from sqlalchemy import create_engine
engine = create_engine(‘sqlite:///example.db‘)
Layer 5: Deployment—Bring It to the World
Finally, deploy your finished project. Use platforms like Heroku, or go container-based with Docker for portability.
Conclusion
Becoming a Python Full Stack Developer is an enriching, multi-layered journey. You’ll transition from writing functional scripts to creating immersive, full-scale applications. Remember, every line of code brings you closer to mastering the art of modern development. So, take the leap—your path to innovation starts now.
FAQ's
The process of building an application that is to run on a website and comprises of the development of both the front end (what the users is exposed to) and the back end (the server side logic).
Python language is used in Full Stack Development because it is beginner friendly, have strong frameworks such as Django and Flask and also supports the feature of fast prototyping.
Yes, in essence as a Full Stack Developer you should be aware of how HTML and CSS can be used in designing the aesthetics of web applications.
Database stores and manipulates data of web applications. Django and Flask frameworks of python make all the database and integrating tasks easier to handle.
As such, you can host your Python Full Stack application on Heroku, or containerize your application via Docker.
The most famous example is the mobile phone for communication, maps, and mobile money.
You may also like
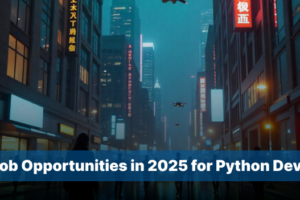
Top 5 Job Opportunities in 2025 for Python Developers
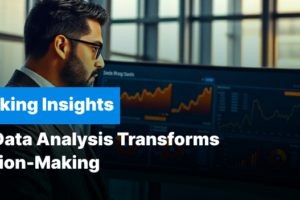